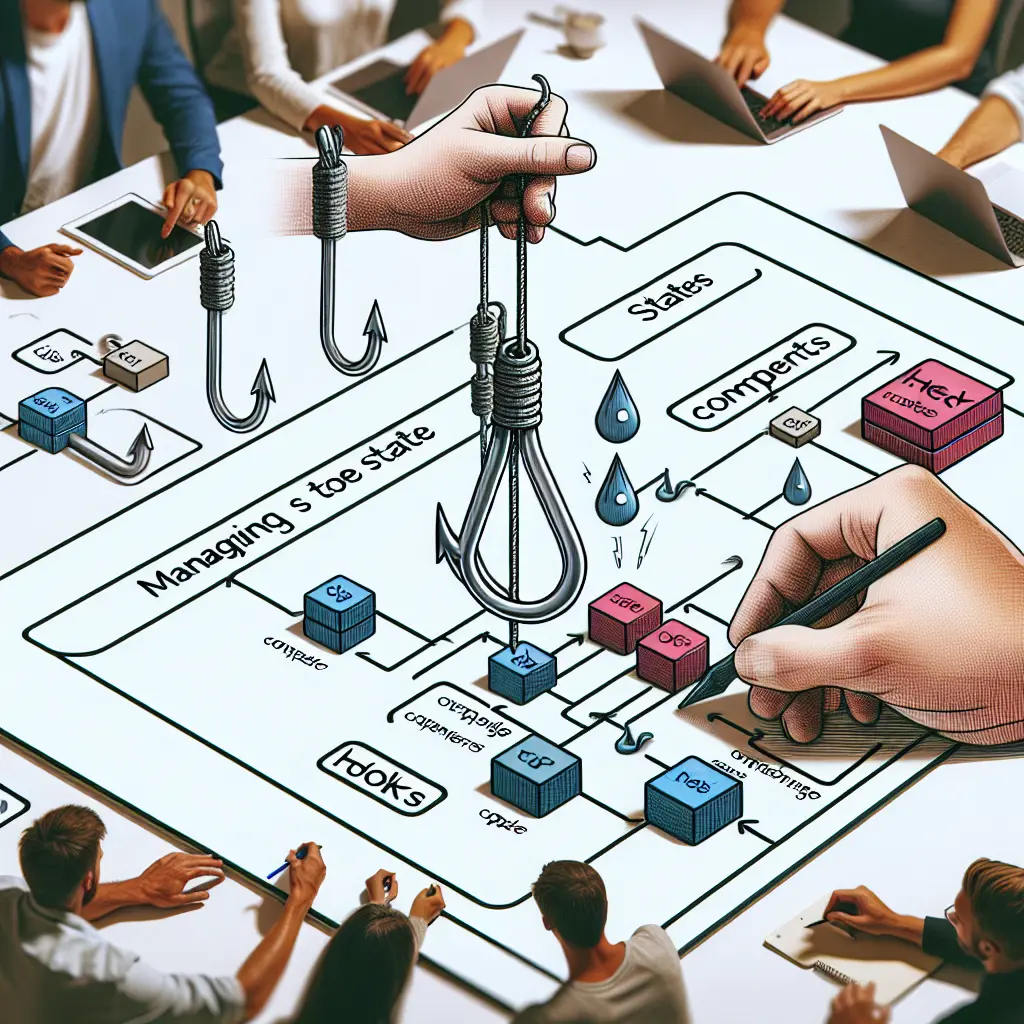
Understanding React Hooks for State Management has revolutionized the way developers build user interfaces using React. Introduced in React 16.8, the Hooks API allows developers to use state and other React features without writing a class, simplifying the code and increasing reusability. This exploration delves into how hooks streamline state management in React functional components, offering practical insights and examples.
React Hooks: A Paradigm Shift
React Hooks offer a suite of functions that let you "hook into" React's state and lifecycle features from function components. This is a significant shift from the traditional class-based approach, aligning with modern JavaScript developments and offering a more readable and maintainable codebase. As developers, embracing this shift is crucial for staying current in the fast-evolving tech landscape.
State Management with useState and useEffect Hooks
The useState
Hook is a fundamental aspect of managing state in React. It lets you add state to functional components, initializing with a state variable and providing a function to update it. This simplicity is vital for React performance optimization, reducing overhead and making state updates straightforward (React Documentation, 2023).
Paired with useState
, the useEffect
Hook replaces several lifecycle methods, allowing side effects in functional components. It runs after every render by default but can be customized using useEffect dependencies to run only when certain values change, optimizing performance by preventing unnecessary operations.
Beyond Basics: useReducer and useContext Hooks
For more complex state logic, the useReducer
Hook offers an alternative to useState. It's particularly useful when the next state depends on the previous one or when managing multiple sub-values of state. This hook simplifies component logic, making it easier to manage large states cohesively.
Meanwhile, the useContext
Hook allows you to manage global state across many components. It helps avoid prop-drilling from parent to child, instead using a Provider/Consumer pattern making state accessible throughout the component tree. This is particularly useful in large applications where state needs to be accessed by many components at different nesting levels.
Crafting Solutions with Custom Hooks
Custom Hooks are a powerful feature for extracting component logic into reusable functions. By creating your own hooks, you can share logic across multiple React components or even across projects. This not only helps in keeping your code DRY but also encapsulates and manages related logic in a scalable way.
React Hooks Best Practices
To harness the full potential of React Hooks, certain best practices are recommended:
- Only Call Hooks at the Top Level: Ensure hooks are called in the same order each time a component renders.
- Use Multiple useState/Effect for Unrelated Logic: Separate different pieces of state into multiple state variables and effects.
- Capitalize on useEffect Dependencies: Effectively managing dependencies ensures your effects run at the right time, not more often than needed.
React Component Lifecycle and Hooks
Understanding the lifecycle of a React component is crucial when using hooks effectively. The useEffect Hook, for instance, encompasses the capabilities of componentDidMount, componentDidUpdate, and componentWillUnmount lifecycle methods from class components, giving you a unified API to handle side effects.
React Hooks Examples and Tutorials
For those new to hooks or needing to deepen their understanding, numerous resources and tutorials are available. The official React Hooks tutorial (Reactjs.org) is a great starting place. For complex scenarios, looking into detailed examples where hooks manage an application's state or control component side effects would be beneficial.
Recent News Impact on React Development
The migration of Coinbase’s 56M users to React Native highlights the robustness and scalability of using React in large applications (TechCrunch, 2023). This move underscores the importance of understanding modern React paradigms like hooks for future-proofing your development skills.
Conclusion
React Hooks simplify managing state and side effects in functional components across both small and large applications. They encourage clean code practices, improve component reusability, and optimize performance. As the tech world reacts to new developments—like Coinbase’s big migration or updates in mobile interactions (CNET, 2023; WhatsApp updates)—staying adept with React and its evolving ecosystem is more crucial than ever.
By leveraging hooks correctly—honoring best practices and continuously exploring advanced patterns like custom hooks—you ensure that your applications are efficient, maintainable, and scalable.
Thank you for joining me in this deep dive into React Hooks for State Management. Keep experimenting, keep learning, and most importantly, keep coding!
Adrianne Blake